Note
Go to the end to download the full example code.
Fitting models without motion correction#
This example illustrates how mdreg can be used to fit models without motion correction. The idea is illustrated for a 3D time series with variable flip angles (VFA), and a linear signal model fit.
Setup#
Perform model fit#
The linear VFA signal model is included in mdreg
as the function
mdreg.fit_spgr_vfa_lin
, which requires the flip angle (FA) values in
degrees as input:
Fitting VFA model: 0%| | 0/269568 [00:00<?, ?it/s]
Fitting VFA model: 2%|█▊ | 6509/269568 [00:00<00:04, 57118.07it/s]
Fitting VFA model: 5%|███▌ | 13222/269568 [00:00<00:04, 59586.27it/s]
Fitting VFA model: 7%|█████▎ | 19878/269568 [00:00<00:04, 59780.59it/s]
Fitting VFA model: 10%|███████ | 26658/269568 [00:00<00:04, 59854.62it/s]
Fitting VFA model: 12%|████████▋ | 32645/269568 [00:00<00:03, 59798.49it/s]
Fitting VFA model: 14%|██████████▎ | 38626/269568 [00:00<00:03, 59455.26it/s]
Fitting VFA model: 17%|███████████▉ | 44571/269568 [00:00<00:03, 59385.28it/s]
Fitting VFA model: 19%|█████████████▋ | 51350/269568 [00:00<00:03, 59164.72it/s]
Fitting VFA model: 21%|███████████████▎ | 57342/269568 [00:00<00:03, 59374.79it/s]
Fitting VFA model: 23%|████████████████▉ | 63282/269568 [00:01<00:03, 59059.52it/s]
Fitting VFA model: 26%|██████████████████▋ | 69898/269568 [00:01<00:03, 59435.18it/s]
Fitting VFA model: 28%|████████████████████▍ | 76664/269568 [00:01<00:03, 60193.24it/s]
Fitting VFA model: 31%|██████████████████████ | 82826/269568 [00:01<00:03, 58921.73it/s]
Fitting VFA model: 33%|███████████████████████▊ | 89073/269568 [00:01<00:03, 59551.09it/s]
Fitting VFA model: 36%|█████████████████████████▋ | 96166/269568 [00:01<00:02, 60127.32it/s]
Fitting VFA model: 38%|██████████████████████████▉ | 102251/269568 [00:01<00:02, 60193.20it/s]
Fitting VFA model: 40%|████████████████████████████▌ | 108621/269568 [00:01<00:02, 59431.94it/s]
Fitting VFA model: 43%|██████████████████████████████▎ | 115202/269568 [00:01<00:02, 59970.74it/s]
Fitting VFA model: 45%|████████████████████████████████▏ | 122409/269568 [00:02<00:02, 60731.67it/s]
Fitting VFA model: 48%|██████████████████████████████████ | 129102/269568 [00:02<00:02, 60871.93it/s]
Fitting VFA model: 50%|███████████████████████████████████▊ | 135877/269568 [00:02<00:02, 61210.39it/s]
Fitting VFA model: 53%|█████████████████████████████████████▌ | 142764/269568 [00:02<00:02, 61739.97it/s]
Fitting VFA model: 56%|███████████████████████████████████████▍ | 149822/269568 [00:02<00:01, 62108.47it/s]
Fitting VFA model: 58%|█████████████████████████████████████████▏ | 156330/269568 [00:02<00:01, 61598.38it/s]
Fitting VFA model: 61%|██████████████████████████████████████████▉ | 163148/269568 [00:02<00:01, 61822.78it/s]
Fitting VFA model: 63%|████████████████████████████████████████████▊ | 169992/269568 [00:02<00:01, 61938.21it/s]
Fitting VFA model: 65%|██████████████████████████████████████████████▍ | 176186/269568 [00:02<00:01, 61872.61it/s]
Fitting VFA model: 68%|████████████████████████████████████████████████ | 182373/269568 [00:03<00:01, 61867.02it/s]
Fitting VFA model: 70%|█████████████████████████████████████████████████▋ | 188559/269568 [00:03<00:01, 61812.87it/s]
Fitting VFA model: 72%|███████████████████████████████████████████████████▎ | 194740/269568 [00:03<00:01, 61715.72it/s]
Fitting VFA model: 75%|█████████████████████████████████████████████████████▏ | 201730/269568 [00:03<00:01, 61317.14it/s]
Fitting VFA model: 77%|██████████████████████████████████████████████████████▊ | 207926/269568 [00:03<00:01, 61472.92it/s]
Fitting VFA model: 79%|████████████████████████████████████████████████████████▍ | 214135/269568 [00:03<00:00, 61635.36it/s]
Fitting VFA model: 82%|██████████████████████████████████████████████████████████▎ | 221225/269568 [00:03<00:00, 61494.17it/s]
Fitting VFA model: 85%|███████████████████████████████████████████████████████████▉ | 227792/269568 [00:03<00:00, 61055.56it/s]
Fitting VFA model: 87%|█████████████████████████████████████████████████████████████▊ | 234591/269568 [00:03<00:00, 61394.70it/s]
Fitting VFA model: 90%|███████████████████████████████████████████████████████████████▌ | 241435/269568 [00:03<00:00, 61754.36it/s]
Fitting VFA model: 92%|█████████████████████████████████████████████████████████████████▍ | 248267/269568 [00:04<00:00, 61971.72it/s]
Fitting VFA model: 95%|███████████████████████████████████████████████████████████████████▏ | 254919/269568 [00:04<00:00, 61676.99it/s]
Fitting VFA model: 97%|████████████████████████████████████████████████████████████████████▉ | 261650/269568 [00:04<00:00, 61639.77it/s]
Fitting VFA model: 100%|██████████████████████████████████████████████████████████████████████▋| 268523/269568 [00:04<00:00, 62002.35it/s]
Fitting VFA model: 100%|███████████████████████████████████████████████████████████████████████| 269568/269568 [00:04<00:00, 60861.84it/s]
divide by zero encountered in divide
Plot the model parameters:
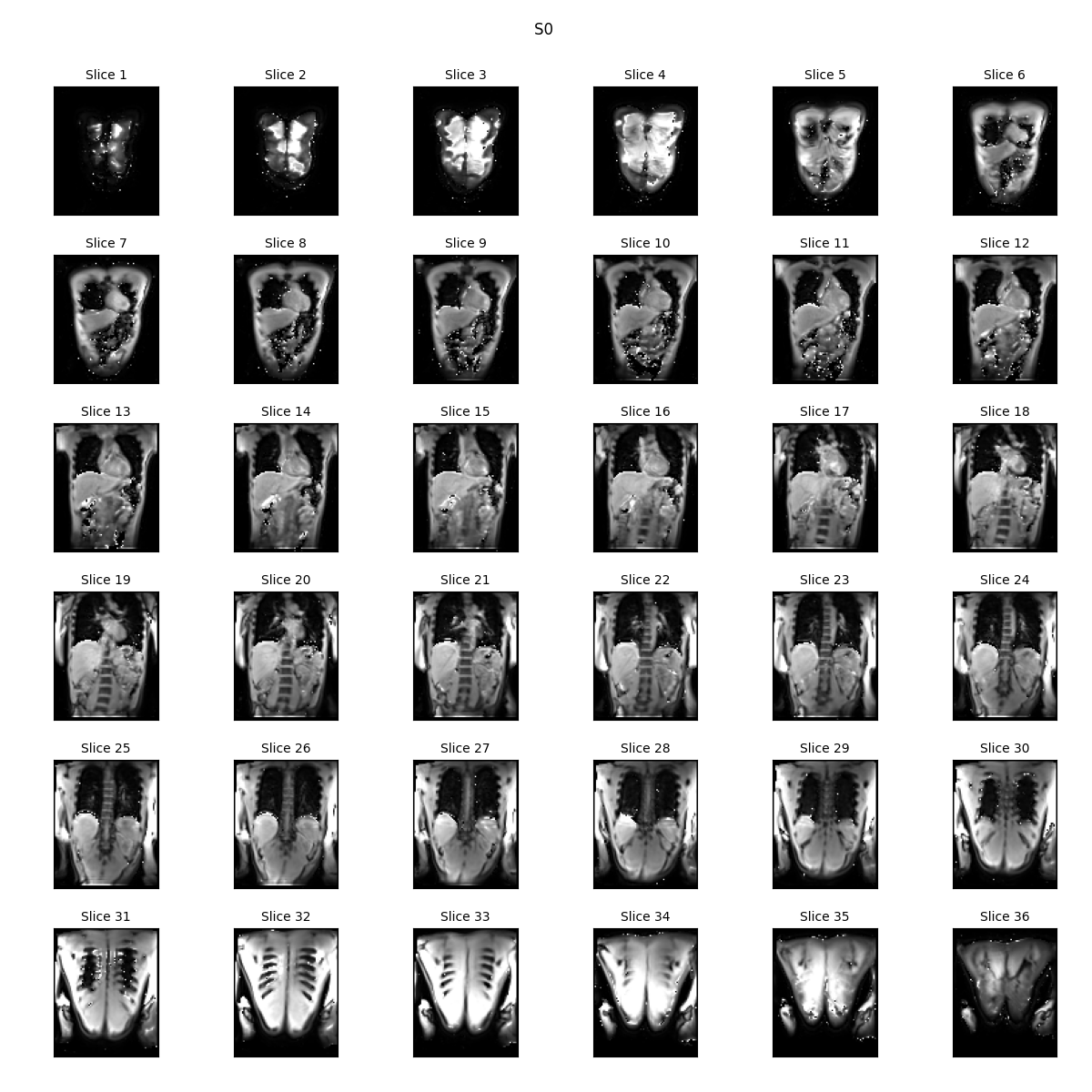
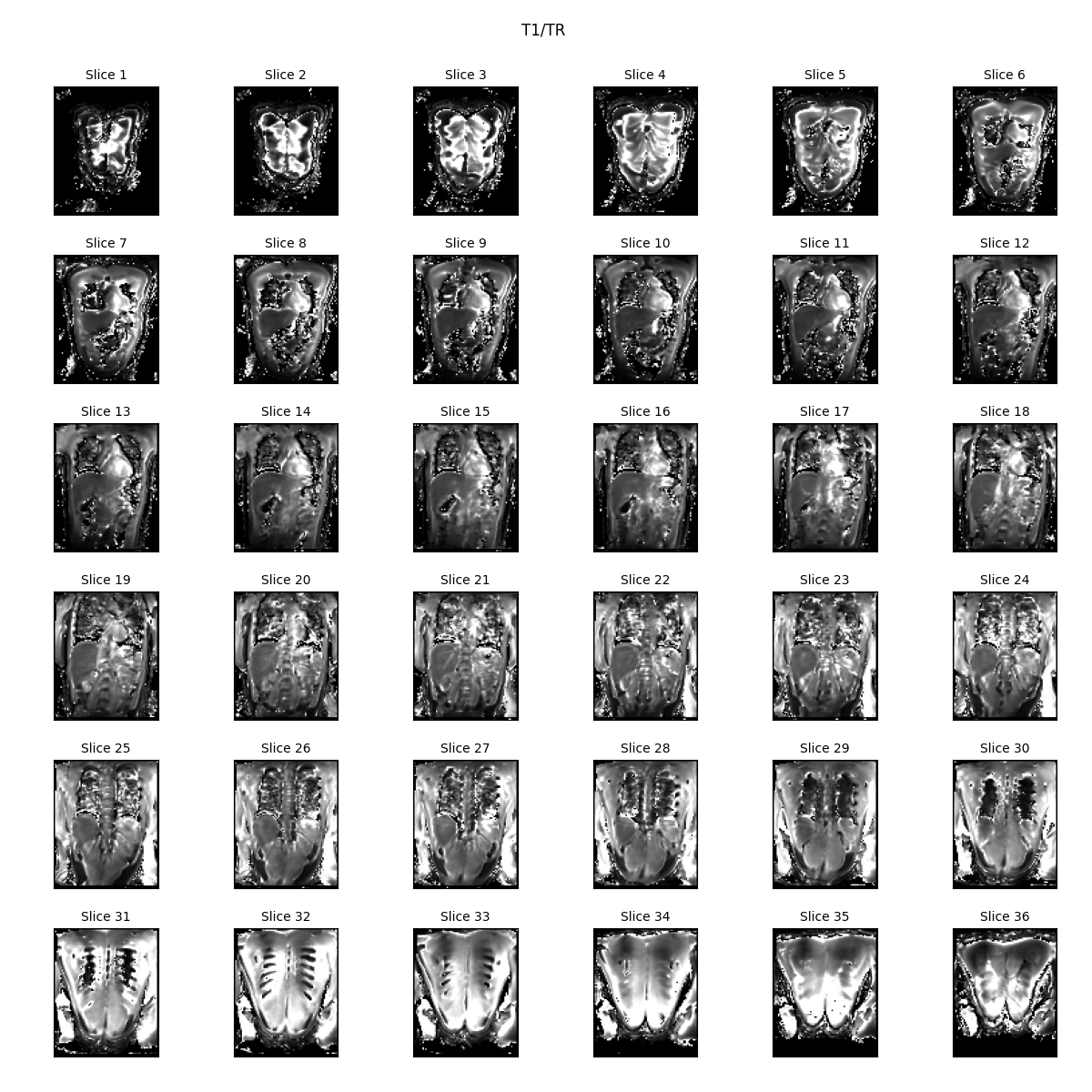
Check the model fit:
anim = mdreg.plot.animation(
fit,
title='VFA model fit',
vmin=0,
vmax=np.percentile(array, 99),
)
Pixel-by-pixel fitting#
Alternatively, the function mdreg.fit_pixels
can be used to fit any
single-pixel model directly:
Check the model fit:
anim = mdreg.plot.animation(
fit,
title='VFA model fit',
vmin=0,
vmax=np.percentile(array, 99),
)
Total running time of the script: (64 minutes 33.466 seconds)